Deposit SDK (Web)
Overview
The Deposit SDK provides a simple way to integrate the Onramp service into your games/dapps. Allow users to purchase cryptocurrencies directly using fiat currencies via different payment methods such as card, bank transfer and other local payment methods through the Ronin Waypoint service.
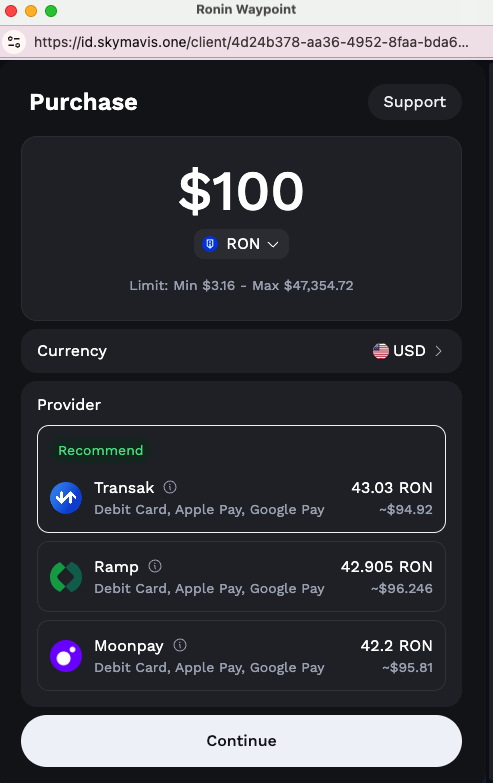
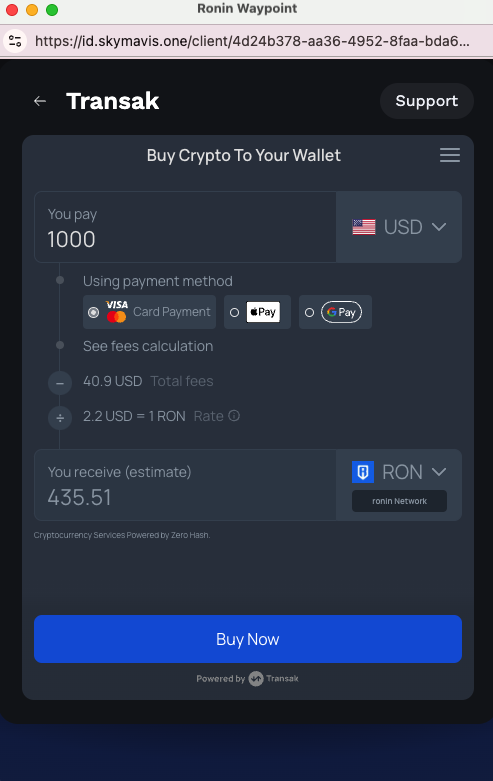
Prerequisites
Permission to use the Ronin Waypoint Account Service. For more information, see Setup and configuration.
Installation
To install the SDK, use the following command:
- npm
- Yarn
- pnpm
npm install @sky-mavis/waypoint
yarn add @sky-mavis/waypoint
pnpm add @sky-mavis/waypoint
Usage
Initialize
import { Deposit } from "@sky-mavis/waypoint/deposit";
const deposit = new Deposit({
clientId: "<client_id>",
});
Parameters for the Deposit
class include:
Field | Required? | Description |
---|---|---|
clientId | Required | The client ID from the Ronin Developer Console. For more information, see Ronin Waypoint settings. |
redirectUri | Optional | Equivalent to the REDIRECT URI configured in Ronin Waypoint settings. Default is window.location.origin . |
theme | Optional | The theme of the deposit modal. Available values are light and dark . |
Open the deposit pop-up
deposit.start();
The start
method also accepts an object with the following parameters to pre-fill the user's information:
Field | Required? | Description |
---|---|---|
walletAddress | Optional | The Ronin wallet address of the customer. |
fiatAmount | Optional | The initial amount of fiat currency you want the customer to buy cryptocurrency. |
fiatCurrency | Optional | The code of the fiat currency you want the customer to buy cryptocurrency, e.g. USD , EUR , VND etc. |
cryptoCurrency | Optional | The code of the cryptocurrency you want the customer to buy, e.g RON , SLP , AXS etc. |
email | Optional | The email that will be used to identify your customer and their order. |
In the Ramp provider, when a user enters their wallet address manually, the address must include the ronin:
prefix. For example, ronin:1234567890abcdef1234567890abcdef12345678
.
The start
method returns a Promise
that resolves with an object containing the transaction details.
Field | Description |
---|---|
provider | The provider used for the transaction. |
transactionHash | The hash of the transaction. |
fiatCurrency | The fiat currency used in the transaction. |
cryptoCurrency | The cryptocurrency used in the transaction. |
fiatAmount | The amount of fiat currency involved in the transaction. |
cryptoAmount | The amount of cryptocurrency involved in the transaction. |
If the transaction is failed or cancelled, the Promise
will reject with an object containing the error details.
Field | Description |
---|---|
code | The error code indicating the type of error. |
message | The reason for the error or cancellation. |
The deposit error codes are as follows:
Code | Description |
---|---|
4001 | The user has closed the deposit pop-up. |
-32603 | The deposit transaction has failed. |
Sample code
Here is an example of how to implement Onramp service in your application:
Step 1: Initialize an instance from the Deposit
class
import {Deposit} from '@sky-mavis/waypoint/deposit';
export const deposit = new Deposit({
clientId: '4d24b378-aa36-4952-8faa-bda63c9a4932',
});
Step 2: Open the deposit pop-up
function StartDeposit() { const [result, setResult] = useState(null); const handleDeposit = async () => { try { const result = await deposit.start({ fiatCurrency: 'USD', cryptoCurrency: 'RON', fiatAmount: 100, }); setResult(result); } catch (error) { alert(error); } }; return ( <LayoutBox> <Button label="Deposit with Ronin Waypoint" onClick={handleDeposit} /> {result && ( <span>{`You have deposited ${result.cryptoAmount} ${result.cryptoCurrency} to your Wallet`}</span> )} </LayoutBox> ); }